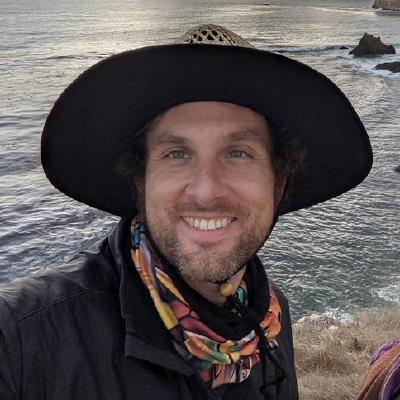
The TLDR pages are a community effort to simplify the beloved man pages with practical examples.
man
is great for in depth understanding of how a command functions, but it isn’t so good for a quick top-level “how the F do I just start using this thing as fast as possible” without having to parse through every flag and detail of the command.tldr
is much better for fast, real-world frequent use cases showcasing a variety of different ways to use the command
Examples
Using the find
command
- Let’s find all
.gif
and.jpg
files in the home folder. We know about thefind
command, but just forgot exactly how to use it.- Assume we’re already in the home folder
~
- Looking at the
man
manual page seems overwhelming. All we want to do is find some real world examples and modify the syntax according to our needs, not understand every detail of how it works. - Let’s take a look at the
tldr
page forfind
instead, and compare it to theman
page output…
- Assume we’re already in the home folder
- After reading through the
tldr find
page, we can easily construct a command to search for all of the files that we want:find . -name "
.gif" -or -name "
.jpg"
Output of tldr find
:
# find
Find files or directories under the given directory tree, recursively.
- Find files by extension:
find root_path -name '*.ext'
- Find files by matching multiple patterns:
find root_path -name '*pattern_1*' -or -name '*pattern_2*'
- Find directories matching a given name, in case-insensitive mode:
find root_path -type d -iname '*lib*'
- Find files matching a path pattern:
find root_path -path '**/lib/**/*.ext'
- Find files matching a given pattern, excluding specific paths:
find root_path -name '*.py' -not -path '*/site-packages/*'
- Find files matching a given size range:
find root_path -size +500k -size -10M
- Run a command for each file (use `{}` within the command to access the filename):
find root_path -name '*.ext' -exec wc -l {} \;
- Find files modified in the last 7 days, and delete them:
find root_path -mtime -7 -delete
- Compare that to the output of
man find
here (spoiler alert: It’s LONG)
Awk
- Using
tldr
to learn how to useawk
-
Using the command from the above example on
find
, we can find out how many GIF and JPG pictures exist. To do this, we’ll need to remove everything but the file extension usingsed
,sort
the text, and pipe it throughuniq -c
to get a count of each filetype. The resulting command looks like this:find . -name "*.gif" -or -name "*.jpg" | sed 's/^.*\.//g' | sort | uniq -c ### OUTPUT ### 266 gif 13165 jpg
-
This is great, but let’s use
awk
to go a step further. Usingtldr
, we can see that you can useawk '{s+=$1} END {print s}' filename
to add up the values in the first column.find . -name "*.gif" -or -name "*.jpg" | sed 's/^.*\.//g' | sort | uniq -c | awk '{s+=$1} END {print "Total Images: "s}'
-
Let’s just add the
awk
command to the piped output of our last command. This will allow us to drop thefilename
parameter. The resulting command and output will look like this:❯ find . -name "*.gif" -or -name "*.jpg" | sed 's/^.*\.//g' | sort | uniq -c | awk '{s+=$1} END {print s}' ### OUTPUT ### 13431
-
Output of tldr awk
:
# awk
A versatile programming language for working on files.
More information: <https://github.com/onetrueawk/awk>.
- Print the fifth column (a.k.a. field) in a space-separated file:
awk '{print $5}' filename
- Print the second column of the lines containing "something" in a space-separated file:
awk '/something/ {print $2}' filename
- Print the last column of each line in a file, using a comma (instead of space) as a field separator:
awk -F ',' '{print $NF}' filename
- Sum the values in the first column of a file and print the total:
awk '{s+=$1} END {print s}' filename
- Sum the values in the first column and pretty-print the values and then the total:
awk '{s+=$1; print $1} END {print "--------"; print s}' filename
- Print every third line starting from the first line:
awk 'NR%3==1' filename
- Print all values starting from the third column:
awk '{for (i=3; i <= NF; i++) printf $i""FS; print""}' filename
- Print different values based on conditions:
awk '{if ($1 == "foo") print "Exact match foo"; else if ($1 ~ "bar") print "Partial match bar"; else print "Baz"}' filename
- Compare to the output of
man awk
, here:
TLDR?
And, yes…you can even tldr tldr!
Output of tldr tldr
:
# tldr
Command-line client for tldr pages.
Displays simplified and community-driven man pages.
More information: <https://tldr.sh>.
- Get typical usages of a command (hint: this is how you got here!):
tldr command
- Show the tar tldr page for Linux:
tldr -p linux tar
- Get help for a git subcommand:
tldr git-checkout
Installation instructions
-
Currently the most mature client is the node.js one, which you can easily install from NPM:
npm install -g tldr
-
For Manjaro / Arch users: I suggest using
npm
to install rather than using theAUR
, because theAUR
version is based on the Python library which is not updated as often as the Node.js client is
Copy of Additional Installation Instructions
Adding colors (using NPM version)
- By default, tldr installed using NPM won’t show any colors. We can fix that by adding a configuration file, with a default theme of “ocean” that was obtained on the website here.
- Create a
~/.tldrrc
file containing the following:
{
"themes": {
"ocean": {
"commandName": "bold, cyan",
"mainDescription": "",
"exampleDescription": "green",
"exampleCode": "cyan",
"exampleToken": "dim"
}
},
"theme": "ocean"
}
- Or to make it easy, just execute this one-liner to automatically create the file:
echo '{
"themes": {
"ocean": {
"commandName": "bold, cyan",
"mainDescription": "",
"exampleDescription": "green",
"exampleCode": "cyan",
"exampleToken": "dim"
}
},
"theme": "ocean"
}' > ~/.tldrrc